Introduction
Authentication bypass is a security vulnerability that allows an attacker to access resources that require authentication without actually authenticating. This vulnerability allows the attacker to gain access to portions of the application or data the target is trying to protect. Depending on the exposed portions, this can be a very critical issue in a web application.
What is Authentication Bypass?
Authentication bypass occurs when an application fails to properly verify user credentials, allowing an attacker to access resources meant for authenticated users. This can happen due to insufficient or missing authentication mechanisms within the application.
Methods of Authentication Bypass
Authentication bypass can be achieved through two main methods: manual access and automated script usage.
Manual Access:
Manual access involves examining the application’s site map and directly accessing the pages listed, rather than following the usual navigation flow. This method includes:
- Accessing pages using a browser
- Inspecting and manipulating URL parameters
- Using keys and IDs to gain access
Automated Script Usage:
Automated scripts make exploiting this flaw easier. Scripts can brute force directory and file names to find valid filenames that allow access. This method involves:
- Writing scripts to brute force IDs and keys
- Guessing page names through brute force to find accessible pages
Detecting Authentication Bypass Vulnerability
When a security tester finds an authentication bypass issue, they need to determine what the vulnerability provides access to. Does it grant access to the entire site or just a portion of it? Understanding this is crucial to assess the severity of the vulnerability.
Conclusion
Authentication bypass is a serious security flaw that allows attackers to access unauthorized areas within an application. Both manual and automated methods can be used to exploit these vulnerabilities, significantly compromising the security of the application. Identifying and addressing such flaws is critical to maintaining application security.
Flask Application Demonstrating Authentication Bypass Vulnerability
In this section, we will create a simple Flask application with an authentication bypass vulnerability. This application is for educational purposes only and is not recommended for use in production environments.
Install Required Libraries
To install Flask, use the following command:
pip install flask
Create the Flask Application
Copy the following code into a file named app.py
. This code demonstrates a simple Flask application with an authentication bypass vulnerability.
from flask import Flask, request, redirect, url_for, session, render_template
app = Flask(__name__)
app.secret_key = 'supersecretkey'users = {
'admin': 'password123',
'user': 'userpass'
}@app.route('/')
def home():
if 'username' in session:
return render_template('home.html', username=session['username'])
return render_template('home.html', username=None)@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
if username in users and users[username] == password:
session['username'] = username
return redirect(url_for('home'))
return render_template('login.html', error=True)
return render_template('login.html', error=False)@app.route('/logout')
def logout():
session.pop('username', None)
return redirect(url_for('home'))@app.route('/admin')
def admin():
return render_template('admin.html')if __name__ == '__main__':
app.run(debug=True)
HTML Templates
Create a directory named templates
and inside it, create the following files:
- base.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>{% block title %}Flask App{% endblock %}</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
</head>
<body>
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="{{ url_for('home') }}">FlaskApp</a>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav ml-auto">
{% if username %}
<li class="nav-item">
<a class="nav-link" href="#">{{ username }}</a>
</li>
<li class="nav-item">
<a class="nav-link" href="{{ url_for('logout') }}">Logout</a>
</li>
{% else %}
<li class="nav-item">
<a class="nav-link" href="{{ url_for('login') }}">Login</a>
</li>
{% endif %}
</ul>
</div>
</nav>
<div class="container mt-4">
{% block content %}{% endblock %}
</div>
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.5.2/dist/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</body>
</html>
- home.html
{% extends "base.html" %}
{% block title %}Home{% endblock %}
{% block content %}
<div class="jumbotron">
<h1 class="display-4">Welcome{% if username %}, {{ username }}{% endif %}!</h1>
{% if username %}
<p class="lead">You are logged in. <a href="{{ url_for('logout') }}" class="btn btn-primary btn-lg">Logout</a></p>
{% else %}
<p class="lead">You are not logged in. <a href="{{ url_for('login') }}" class="btn btn-primary btn-lg">Login</a></p>
{% endif %}
</div>
{% endblock %}
- login.html
{% extends "base.html" %}
{% block title %}Login{% endblock %}
{% block content %}
<h2>Login</h2>
{% if error %}
<div class="alert alert-danger" role="alert">
Invalid credentials. Please try again.
</div>
{% endif %}
<form method="post">
<div class="form-group">
<label for="username">Username</label>
<input type="text" class="form-control" id="username" name="username" required>
</div>
<div class="form-group">
<label for="password">Password</label>
<input type="password" class="form-control" id="password" name="password" required>
</div>
<button type="submit" class="btn btn-primary">Login</button>
</form>
{% endblock %}
- admin.html
{% extends "base.html" %}
{% block title %}Admin{% endblock %}
{% block content %}
<h2>Admin Page</h2>
<p>Welcome to the admin page. <a href="{{ url_for('home') }}" class="btn btn-secondary">Home</a></p>
{% endblock %}h
Run the Application
Run the following command in your terminal to start the appa
python app.py
Test the Application
Open your browser and navigate to http://127.0.0.1:5000
to test your modernized application. Try accessing the /admin
page directly by entering http://127.0.0.1:5000/admin
in the browser. You will notice that you can access this page without logging in.
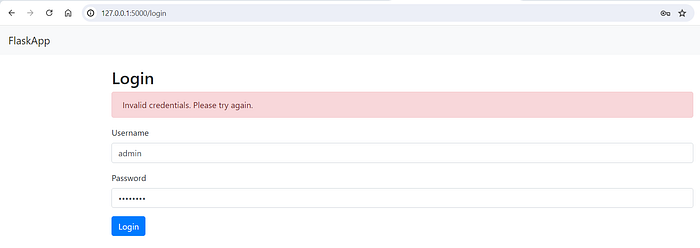
